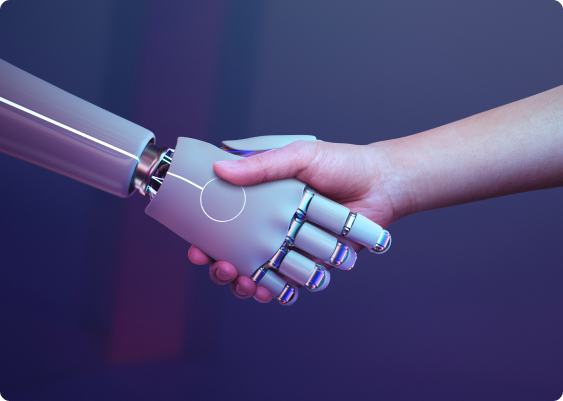
Lamzing
February 16, 2023
React JS in TypeScript
ReactJS is a popular library for building user interfaces, and TypeScript is a type-safe language that helps catch errors before they happen. Together, they make a powerful combination for creating robust and scalable web applications.
TypeScript is a superset of JavaScript, which means that any valid JavaScript code is also valid TypeScript code. However, TypeScript adds additional features such as static typing and interfaces that help catch errors at compile time rather than at runtime.
With static typing, developers can define the shape of props and state using interfaces, which can then be used to enforce type safety. This helps catch errors early in the development process and prevents unexpected bugs from occurring in production.
Getting started with ReactJS in TypeScript:
To get started with ReactJS in TypeScript, there are a few steps to follow:
- Install TypeScript - You can install TypeScript using npm or yarn. Here is the command to install TypeScript using npm:
- npm install typescript --save-dev
- Create a new React app - You can create a new React app using the create-react-app command-line tool. Here is the command to create a new React app in TypeScript:
- npx create-react-app my-app --template typescript
- Write your code - You can now write your React components using TypeScript.
Here's an example of using TypeScript with ReactJS:
import React, { FC } from 'react';
interface Props {
name: string;
age: number;
}
const MyComponent: FC<Props> = ({ name, age }) => {
return (
<div>
<p>Name: {name}</p>
<p>Age: {age}</p>
</div>
);
};
In this example, we define an interface Props that specifies the shape of the props object that our component expects. We then use this interface to define the type of our component using the FC (Function Component) type from React. This ensures that the props passed to our component are of the correct type, and any errors related to props are caught at compile time.
Another benefit of using TypeScript with ReactJS is that it provides better tooling support. IDEs such as Visual Studio Code can provide better code completion and error checking, making it easier to write and maintain code.
In summary, using TypeScript with ReactJS can provide several benefits, including better type safety, catching errors at compile time, and improved tooling support. If you're starting a new ReactJS project, consider using TypeScript to improve the quality and maintainability of your code.
Benefits of ReactJS in TypeScript
- Type Safety: TypeScript adds static typing to your code, allowing you to catch errors at compile-time instead of run-time. This can help catch bugs early in the development process, reducing the time and effort required for testing and debugging.
- Improved Code Quality: By adding type annotations to your code, TypeScript can help ensure that your code is consistent and well-structured. This can improve readability and maintainability, making it easier for other developers to work with your code.
- Enhanced Productivity: TypeScript's tooling can help improve developer productivity by providing better auto-completion, code navigation, and refactoring tools. This can save time and effort when writing and maintaining your code base.
- Better code organization - TypeScript provides a way to define interfaces and types, which helps in better organizing the code. It improves readability, maintainability, and refactoring of code.
- Improved developer experience - With TypeScript, developers can take advantage of better auto-completion, code navigation, and inline documentation. It can also help prevent common errors that occur in JavaScript projects.
- Easier codebase management - In larger codebases, TypeScript can help developers better manage the complexity of the code. It can also help in better code reuse and sharing between different components.
Best Practices for Using ReactJS in TypeScript
- Use React.FC: Use the React.FC type to define your functional components. This will provide better type checking for your props and ensure that your components are compatible with the rest of the React ecosystem.
- Define Props with Interfaces: Use interfaces to define the shape of your component's props. This will provide better type checking and ensure that your components are used correctly.
- Use Generics: Use generics to create reusable components and HOCs. This can reduce code duplication and improve code maintainability.
- Use Enums: Use enums to define constants that are used in your application. This can help make your code more readable and easier to maintain.
- Use React Hooks: Use React Hooks to manage state and side-effects in your components. This can improve code readability and reduce complexity.
Conclusion:
Using ReactJS in TypeScript can provide significant benefits in terms of code quality, type safety, and developer productivity. By following best practices such as using React.FC, interfaces, generics, enums, and React Hooks, you can create maintainable and high-quality code. If you're new to TypeScript or ReactJS, it may take some time to get used to the syntax and tools, but the investment can pay off in the long run.